Reading and Storing Names in an Array From User Input C
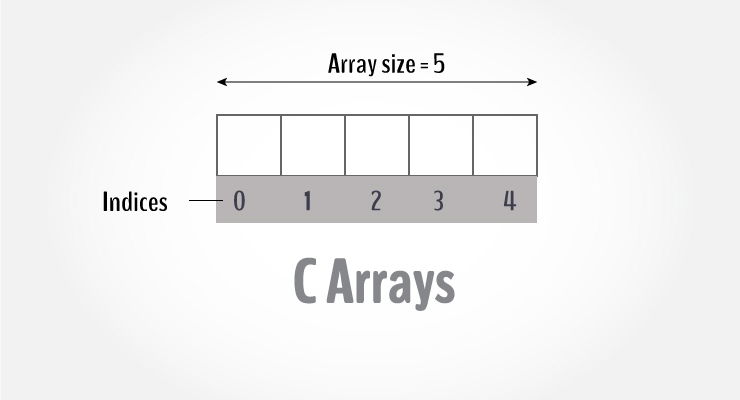
An array is a variable that tin store multiple values. For example, if you want to store 100 integers, you tin can create an assortment for it.
int data[100];
How to declare an array?
dataType arrayName[arraySize];
For instance,
float mark[5];
Hither, we declared an array, mark, of floating-point blazon. And its size is 5. Meaning, it can hold 5 floating-point values.
Information technology'southward important to notation that the size and blazon of an array cannot be changed once it is declared.
Admission Array Elements
Y'all can access elements of an array by indices.
Suppose you alleged an assortment mark equally above. The starting time element is mark[0], the second chemical element is mark[1] and so on.
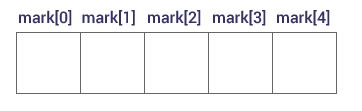
Few keynotes:
- Arrays have 0 every bit the starting time index, not ane. In this example, mark[0] is the get-go element.
- If the size of an array is north, to access the last element, the
due north-1
index is used. In this instance, mark[4] - Suppose the starting address of
mark[0]
is 2120d. Then, the address of themark[1]
will be 2124d. Similarly, the accost ofmark[2]
will be 2128d and and so on.
This is considering the size of afloat
is four bytes.
How to initialize an array?
It is possible to initialize an assortment during proclamation. For instance,
int mark[five] = {19, 10, 8, 17, 9};
You can also initialize an array like this.
int mark[] = {xix, 10, eight, 17, 9};
Hither, we haven't specified the size. However, the compiler knows its size is 5 equally we are initializing information technology with 5 elements.
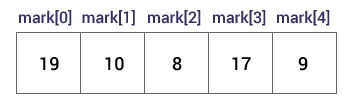
Here,
marking[0] is equal to 19 marking[one] is equal to x marker[ii] is equal to eight marking[three] is equal to 17 marker[4] is equal to 9
Modify Value of Array elements
int marker[5] = {nineteen, 10, 8, 17, 9} // make the value of the tertiary chemical element to -1 mark[ii] = -1; // make the value of the fifth element to 0 mark[4] = 0;
Input and Output Assortment Elements
Here'southward how you can take input from the user and store it in an array element.
// have input and store it in the 3rd element scanf("%d", &mark[2]); // accept input and store it in the ith element scanf("%d", &marking[i-one]);
Here's how you can print an private element of an array.
// print the first element of the array printf("%d", marking[0]); // print the third element of the array printf("%d", mark[2]); // print ith element of the assortment printf("%d", mark[i-1]);
Example 1: Array Input/Output
// Programme to have 5 values from the user and store them in an array // Print the elements stored in the assortment #include <stdio.h> int principal() { int values[5]; printf("Enter 5 integers: "); // taking input and storing it in an array for(int i = 0; i < v; ++i) { scanf("%d", &values[i]); } printf("Displaying integers: "); // printing elements of an array for(int i = 0; i < five; ++i) { printf("%d\n", values[i]); } return 0; }
Output
Enter 5 integers: i -3 34 0 three Displaying integers: 1 -3 34 0 3
Here, we take used afor
loop to take five inputs from the user and shop them in an assortment. Then, using some otherfor
loop, these elements are displayed on the screen.
Example 2: Summate Average
// Plan to discover the average of n numbers using arrays #include <stdio.h> int main() { int marks[10], i, n, sum = 0, average; printf("Enter number of elements: "); scanf("%d", &n); for(i=0; i < n; ++i) { printf("Enter number%d: ",i+1); scanf("%d", &marks[i]); // adding integers entered by the user to the sum variable sum += marks[i]; } average = sum / n; printf("Average = %d", boilerplate); return 0; }
Output
Enter n: five Enter number1: 45 Enter number2: 35 Enter number3: 38 Enter number4: 31 Enter number5: 49 Boilerplate = 39
Here, we accept computed the average of n numbers entered by the user.
Access elements out of its bound!
Suppose y'all alleged an array of ten elements. Allow'due south say,
int testArray[ten];
You lot can admission the array elements from testArray[0]
to testArray[9]
.
Now let's say if you try to admission testArray[12]
. The element is not available. This may cause unexpected output (undefined behavior). Sometimes you might go an mistake and some other time your programme may run correctly.
Hence, yous should never access elements of an array outside of its bound.
Multidimensional arrays
In this tutorial, you learned about arrays. These arrays are called ane-dimensional arrays.
In the next tutorial, yous volition learn nearly multidimensional arrays (array of an array).
Source: https://www.programiz.com/c-programming/c-arrays